Creating widgets for BlogEngine.NET 1.4
The new BlogEngine.NET 1.4 let’s you easily create and distribute widgets like the ones you see on the right hand side on this page. Widgets are basically just small pieces of content and you can leverage the entire .NET Framework to create them. They can pull data from BlogEngine.NET itself or from external sources like web services or remote XML files. Everything is allowed and possible.
Create a widget
In this guide we will create a widget called Most comments and you can see it live on this site where it is called Hall of fame. The widget lists the people who have written most comments in the last x number of days.
You start by creating a new folder in the widgets folder. The name of the folder will be the name of the widget as well. In the new folder you have to add a user control called widget.ascx. If the widget contains settings that the blog owner should be able to change, then you have to add another user control called edit.ascx. It’s important that you name them exactly widget.ascx and edit.ascx.
Let’s start with widget.ascx. This is the user control that displays the widget content on the blog. The first thing you need to do is to change the inheritance of the code-behind file from System.Web.UI.UserControl to WidgetBase. Then you need to override two properties and one method.
The properties only have getters and they look like this.
/// <summary>
/// Gets the name. It must be exactly the same as the folder that contains the widget.
/// </summary>
public override string Name
{
get { return "Most comments"; }
}
/// <summary>
/// Gets wether or not the widget can be edited.
/// <remarks>
/// The only way a widget can be editable is by adding a edit.ascx file to the widget folder.
/// </remarks>
/// </summary>
public override bool IsEditable
{
get { return true; }
}
The method to override is called LoadWidget and you should use it just as you would have used the Page_Load event handler. In other words, this is where you put all the code that needs to run when the widget loads every time a page is requested. If the widget can be edited then this is where you would retrieve the settings that BlogEngine.NET stores for each individual widget. The settings are stored in a simple StringDictionary.
StringDictionary settings = GetSettings();
The widget framework handles the caching of the settings, so you don’t have to worry about that.
Edit a widget
If the property IsEditable on the widget.ascx is set to True, the widget will render an edit link on the top right corner of the widget. When the edit link is clicked, an iframe popup shows up and loads the second user control edit.ascx. From there you can change the settings and save them.
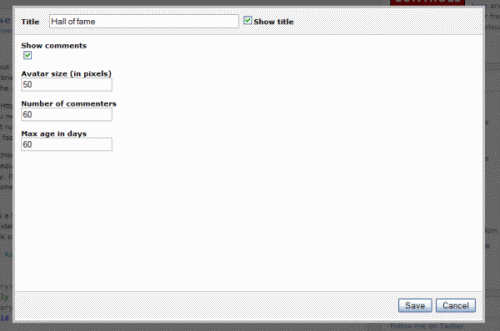
The edit.ascx you create yourself doesn’t handle the title of the widget or whether or not the title show be shown. That is handled by the widget framework for you. The same goes for the save button.
You only have to add the controls needed to change what is specific to your new widget. In the case of the Most comments widget, it’s a checkbox and three text boxes. The first you need to do is to change the inheritance of the code-behind file from System.Web.UI.UserControl to WidgetEditBase. Next you have to override a method called Save, which get’s called by the widget framework when the save button is clicked. It saves the settings entered by the user.
public override void Save()
{
StringDictionary settings = GetSettings();
settings["avatarsize"] = txtSize.Text;
settings["numberofvisitors"] = txtNumber.Text;
settings["days"] = txtDays.Text;
settings["showcomments"] = cbShowComments.Checked.ToString();
SaveSettings(settings);
}
When you bind the settings to the controls, you have to use the Page_Load or similar methods just as you are used to. A good idea is to load the default values if the settings haven’t been set yet.
protected override void OnPreRender(EventArgs e)
{
if (!Page.IsPostBack)
{
txtNumber.Text = "3";
txtSize.Text = "50";
txtDays.Text = "60";
cbShowComments.Checked = true;
StringDictionary settings = GetSettings();
if (settings.ContainsKey("avatarsize"))
txtSize.Text = settings["avatarsize"];
if (settings.ContainsKey("numberofvisitors"))
txtNumber.Text = settings["numberofvisitors"];
if (settings.ContainsKey("days"))
txtNumber.Text = settings["days"];
if (settings.ContainsKey("showcomments"))
cbShowComments.Checked = settings["showcomments"].Equals("true", StringComparison.OrdinalIgnoreCase);
}
}
You have total freedom to create the edit page just as you like with JavaScript, stylesheets or whatever you like. It’s loaded asynchronously when the edit link is clicked and not before.
Download
Download the zip file below and extract the Most comments folder into your widget folder.
Comments
Comments are closed